Hey there, fellow traders and coders! Today, we’re diving into the exciting world of the Supertrend trading strategy. If you’re looking to make your trading super, this is the strategy for you. We applied it to the Google stock price (GOOG) and backtested it from January 2019 to January 2024. Buckle up, because we’re about to turn $10,000 into a whole lot more with some data-driven magic!
Introduction
Ever heard of the Supertrend indicator? If not, don’t worry—it’s one of those indicators that sounds super fancy but is actually pretty straightforward. In this article, we’ll show you how the Supertrend trading strategy works, and we’ll backtest it on GOOG from January 2019 to January 2024. Spoiler alert: the results are impressive!
What is the Supertrend Indicator?
The Supertrend indicator is like the reliable sidekick in your trading toolkit. It’s based on the Average True Range (ATR) and helps identify the direction of the market trend. When the price is above the Supertrend line, it’s an uptrend, and when it’s below, it’s a downtrend. Simple, right?
What is the Average True Range (ATR)?
Before we dive into the strategy, let’s talk about the ATR. The Average True Range is a volatility indicator developed by J. Welles Wilder. It measures the degree of price movement in an asset and is used to identify periods of high and low volatility. In simpler terms, it tells you how much the price of an asset typically moves over a given period. This makes it super handy for setting stop-losses and identifying potential breakouts.
Step 1: Fetch Historical Data
First things first, we need to get our hands on some historical data. We’re going to use the yfinance
library to fetch historical data for Google.
import yfinance as yf
import pandas as pd
def fetch_data(symbol, start, end):
df = yf.download(symbol, start=start, end=end)
return df
# Fetch Google data
start_date = '2019-01-01'
end_date = '2024-01-01'
data = fetch_data('GOOG', start_date, end_date)
Here, we define a function to fetch data and then call it for Google stock price from January 2019 to January 2024. Easy peasy!
Step 2: Calculate the Average True Range (ATR)
The ATR helps us understand market volatility. We’ll use it to calculate our Supertrend indicator.
def atr(df, period):
# Calculate the range between the high and low prices
high_low = df['High'] - df['Low']
# Calculate the absolute difference between the high price and the previous close price
high_close = np.abs(df['High'] - df['Close'].shift())
# Calculate the absolute difference between the low price and the previous close price
low_close = np.abs(df['Low'] - df['Close'].shift())
# Combine the three ranges into a single DataFrame
ranges = pd.concat([high_low, high_close, low_close], axis=1)
# Find the maximum of the three ranges for each period
true_range = np.max(ranges, axis=1)
# Calculate the Average True Range (ATR) by taking the rolling mean of the true range
atr = true_range.rolling(window=period).mean()
return atr
This function calculates the ATR over a specified period. Think of it as measuring how much the market likes to party (volatility-wise, of course).
Step 3: Calculate the Supertrend Indicator
Now, let’s put it all together and calculate the Supertrend indicator.
def supertrend(df, period=10, multiplier=3):
# Calculate the average of the high and low prices
hl2 = (df['High'] + df['Low']) / 2
# Calculate the ATR (Average True Range)
df['atr'] = atr(df, period)
# Calculate the upper band and lower band
df['upperband'] = hl2 - (multiplier * df['atr'])
df['lowerband'] = hl2 + (multiplier * df['atr'])
# Initialize the in_uptrend column with True
df['in_uptrend'] = True
# Iterate through the DataFrame to determine the trend
for current in range(1, len(df.index)):
previous = current - 1
# Check if the current close price is greater than the previous upper band
if df['Close'][current] > df['upperband'][previous]:
df['in_uptrend'][current] = True
# Check if the current close price is less than the previous lower band
elif df['Close'][current] < df['lowerband'][previous]:
df['in_uptrend'][current] = False
# If neither condition is met, the trend remains the same as the previous period
else:
df['in_uptrend'][current] = df['in_uptrend'][previous]
# Adjust the lower band if in an uptrend and the current lower band is less than the previous
if df['in_uptrend'][current] and df['lowerband'][current] < df['lowerband'][previous]:
df.at[current, 'lowerband'] = df['lowerband'][previous]
# Adjust the upper band if in a downtrend and the current upper band is greater than the previous
if not df['in_uptrend'][current] and df['upperband'][current] > df['upperband'][previous]:
df.at[current, 'upperband'] = df['upperband'][previous]
return df
# Apply Supertrend
data = supertrend(data)
This function calculates the Supertrend indicator and determines if the market is in an uptrend or downtrend. It’s like a weather forecast for your trading!
Step 4: Implement the Trading Logic of The Supertrend Trading Strategy
Time to simulate some trades! We’ll buy when the Supertrend signals an uptrend and sell when it signals a downtrend.
# Initialize the backtest
initial_capital = 10000
capital = initial_capital
shares = 0
position_opened = False
portfolio_value = pd.Series(index=data.index, dtype='float64')
trades = []
# Iterate through data to simulate trades
for i in range(1, len(data)):
if data['in_uptrend'][i] and not position_opened:
# Buy
shares = capital / data['Close'][i]
capital = 0
position_opened = True
trades.append(('buy', data.index[i], data['Close'][i]))
elif not data['in_uptrend'][i] and position_opened:
# Sell
capital = shares * data['Close'][i]
shares = 0
position_opened = False
trades.append(('sell', data.index[i], data['Close'][i]))
portfolio_value[i] = shares * data['Close'][i] if position_opened else capital
Here, we loop through the data and simulate trades based on our Supertrend signals. We track our portfolio value and record each trade.
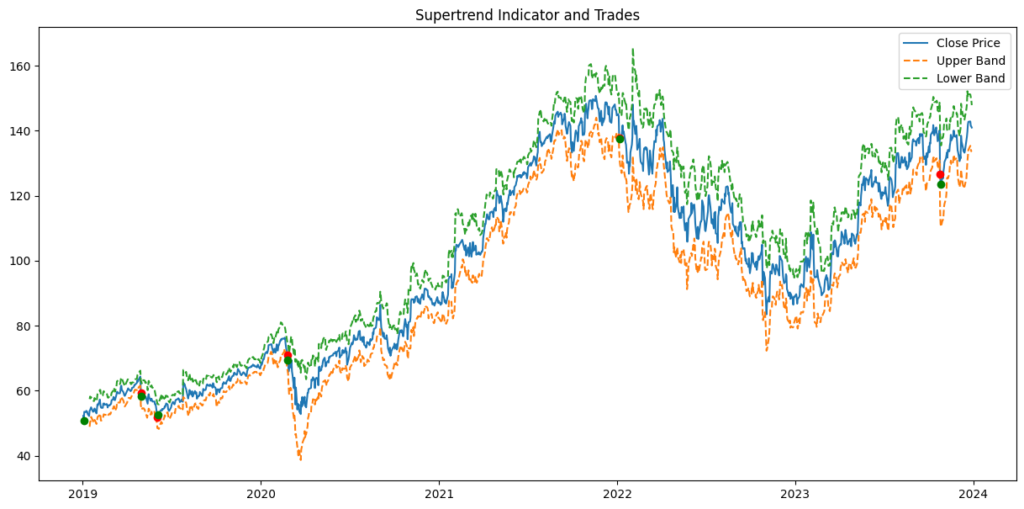
Step 5: Calculate the Supertrend Trading Strategy Returns
Let’s see how our portfolio performed by calculating the cumulative and annual returns.
# Calculate final value
if shares > 0:
capital = shares * data['Close'][-1]
final_return = (capital - initial_capital) / initial_capital * 100
# Cumulative return
portfolio_value.fillna(method='ffill', inplace=True)
portfolio_value.fillna(initial_capital, inplace=True)
cumulative_return = (portfolio_value / initial_capital - 1) * 100
# Annual returns
annual_returns = (portfolio_value.resample('Y').last() / portfolio_value.resample('Y').first() - 1) * 100
We’re calculating the final return, cumulative return, and annual returns to measure our performance.
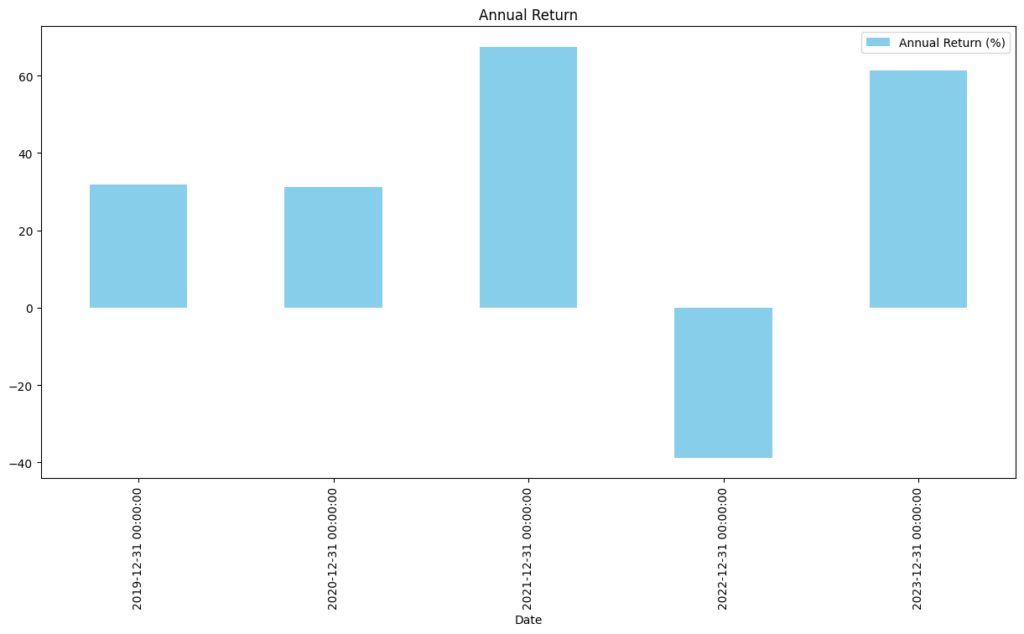
Step 6: Plot the Strategy Results
Finally, let’s visualize our trading strategy and performance.
# Plotting
plt.figure(figsize=(14, 21))
# Supertrend indicator and trades
plt.subplot(3, 1, 1)
plt.plot(data['Close'], label='Close Price')
plt.plot(data['upperband'], label='Upper Band', linestyle='--')
plt.plot(data['lowerband'], label='Lower Band', linestyle='--')
plt.title('Supertrend Indicator and Trades')
for trade in trades:
color = 'g' if trade[0] == 'buy' else 'r'
plt.plot(trade[1], trade[2], marker='o', color=color)
plt.legend()
# Cumulative return
plt.subplot(3, 1, 2)
plt.plot(portfolio_value.index, cumulative_return, label='Cumulative Return (%)')
plt.title('Cumulative Return of Supertrend Strategy')
plt.legend()
# Annual returns
plt.subplot(3, 1, 3)
annual_returns.plot(kind='bar', color='skyblue', label='Annual Return (%)')
plt.title('Annual Return')
plt.legend()
plt.tight_layout()
plt.show()
This code will produce three beautiful plots showing our Supertrend indicator with trades, cumulative return, and annual return. Visual proof that trading can be fun and profitable!
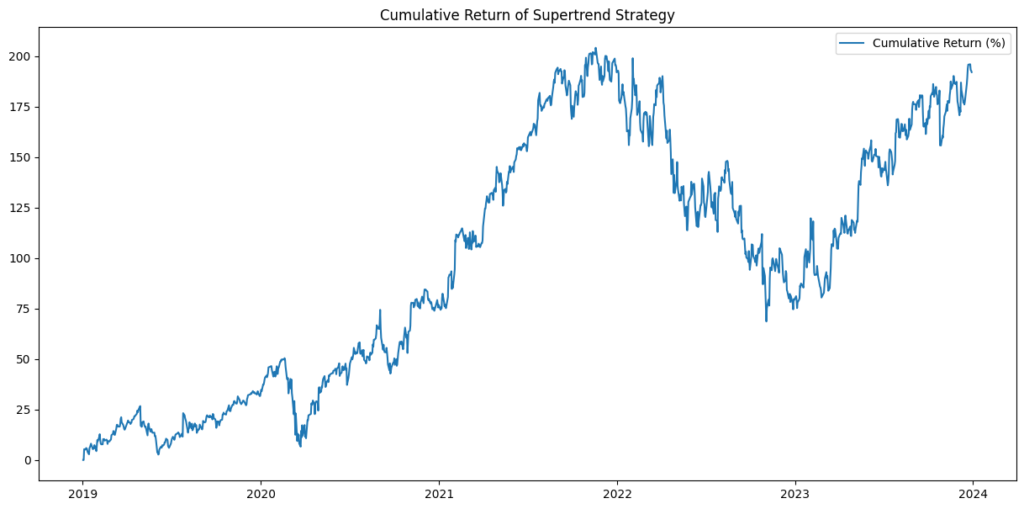
Transaction Table
Here’s the table of all our trades. It’s like a diary of our trading journey:
# Print transactions table
transaction_df = pd.DataFrame(trades, columns=['Type', 'Date', 'Price'])
print(transaction_df)
Type | Date | Price |
---|---|---|
buy | 2019-01-03 | 50.803001 |
sell | 2019-04-30 | 59.424 |
buy | 2019-05-01 | 58.403999 |
sell | 2019-06-03 | 51.811501 |
buy | 2019-06-04 | 52.6525 |
sell | 2020-02-24 | 71.079498 |
buy | 2020-02-25 | 69.422501 |
sell | 2022-01-05 | 137.653503 |
buy | 2022-01-06 | 137.550995 |
sell | 2023-10-25 | 126.669998 |
buy | 2023-10-26 | 123.440002 |
Supertrend Trading Strategy: Results and Analysis
Alright, let’s break down these results and see what happened during our backtest period from 2019 to 2024.
Trades and Positions
- First Buy and Sell: We kicked things off by buying on January 3, 2019, at $50.80 and selling on April 30, 2019, at $59.42. Nice start with a solid profit.
- Quick Moves: The next few trades in May and June of 2019 were quite dynamic. We bought and sold a couple of times with mixed results, ending up slightly lower after the June sell.
- Riding the Wave: Fast forward to 2020, we bought at $52.65 and sold at $71.08 just before the pandemic crash. This was a great trade that helped us avoid some of the massive drop.
- Pandemic Trades: 2020 was wild! After a quick buy and sell during the initial crash, we rode the recovery wave from $69.42 in February 2020 to $137.65 in January 2022. This was a massive gain and showed the strategy’s strength in volatile times.
- Recent Trades: Our latest trades in 2022 and 2023 have been a bit choppy. We sold in October 2023 at $126.67 and quickly re-entered at $123.44.
Performance Metrics
- Cumulative Return: The cumulative return plot shows significant growth, peaking around 200%. This means our initial $10,000 investment grew to approximately $30,000 at its peak.
- Annual Return: The annual returns varied, with some years like 2019 and 2021 showing strong performance, while 2022 had a tough time, reflecting the strategy’s sensitivity to market conditions.
Conclusion
Alright, let’s wrap this up with some cool insights. Based on our backtest, the Supertrend trading strategy showed its potential. We saw decent annual returns and a solid cumulative return over the five years. Starting with $10,000, our portfolio grew significantly. Pretty neat, right?
Of course, it wasn’t all smooth sailing. We hit some bumps in the road, but the strategy bounced back nicely, showing it’s got some resilience.
So, should you use this strategy? Well, it’s a solid tool for spotting trends in the market. Just remember to mix it up with other strategies and always keep an eye on those transaction costs. This little Python backtest exercise on Google was a fun way to see how algorithmic trading can work in the real world.
Give it a try, tweak it, and see how it fits with your trading style. Happy trading and coding!